References
References are currently being uploaded. At the moment, you can only browse a few small VueJS projects.
Latest Photos
In this section you can find the latest photos.
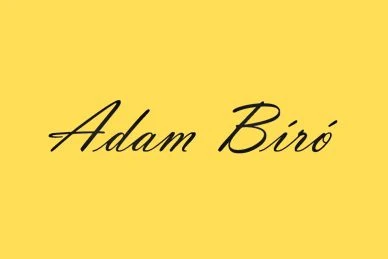
A breathtaking view of the Esztergom Basilica captured from the Slovak side of the Danube River during golden hour. The majestic cathedral dominates the skyline with its impressive dome, surrounded by the historic castle complex and peaceful riverside buildings. Soft, streaky clouds float across the sky, enhancing the dreamy atmosphere of this iconic Hungarian landmark.
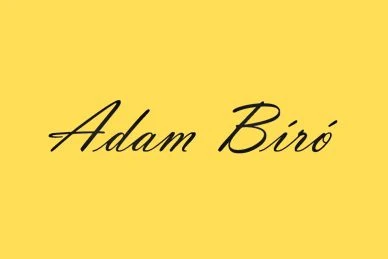
This twilight photograph reveals the moody beauty of Lake Balaton under a soft blue hour sky. With the iconic Badacsony hills in the background and rugged shoreline rocks in the foreground, the scene evokes quiet introspection. The long exposure smooths out the lake surface into a glassy calm, where time seems to slow down.
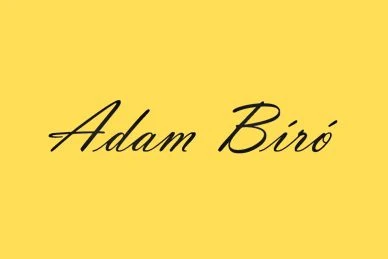
Photographed at sunset on the shores of Lake Balaton, this minimalist composition captures the elegance of bare branches stretching gently into the frame. The calm water mirrors the soft pastels of the sky, creating a tranquil balance between nature’s stillness and delicate light. A serene and contemplative moment in the heart of Hungary.
Latest Blog Posts
Mainly about Programming, PHP, VueJS, Angular, TypeScript or other
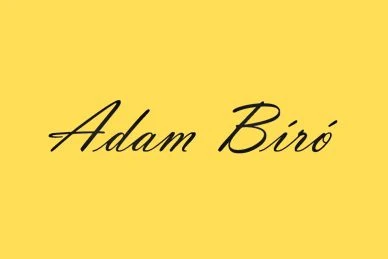
Learn how to avoid overengineering in Vue 3 by managing data locally in your components and embracing its declarative reactive architecture.
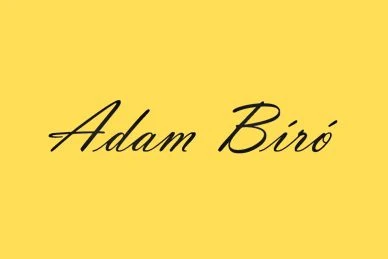
A practical comparison of PHP’s hashing functions including `md5`, `sha1`, `hash()`, `password_hash()`, and `hash_hmac()` — which one should you use and why?
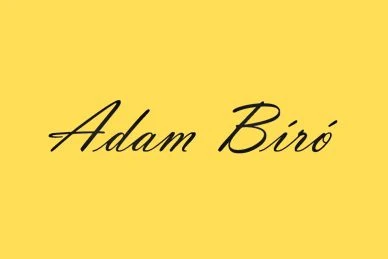
Learn when to use `ref` vs `shallowRef` in Vue 3 and how their reactivity and performance impact your application.
Photo Gallery
In this section you can find the latest photos.